Introduction to Volume Profiles in Python
• 4 minKey Takeaways
- Volume profiles help traders see where the most trading activity occurs at different price levels.
- Learning to compute volume profiles in Python can enhance your data analysis skills.
- This guide will cover basic Python setup, essential libraries, and code examples for volume profile computation.
Introduction
What are Volume Profiles?
Volume profiles are graphical representations that show the trading activity at different price levels over a certain period. Financial analysts use them to identify key support and resistance levels, market sentiment, and trading hotspots. By understanding where trading volume is concentrated, you can gain valuable insights into market behavior.
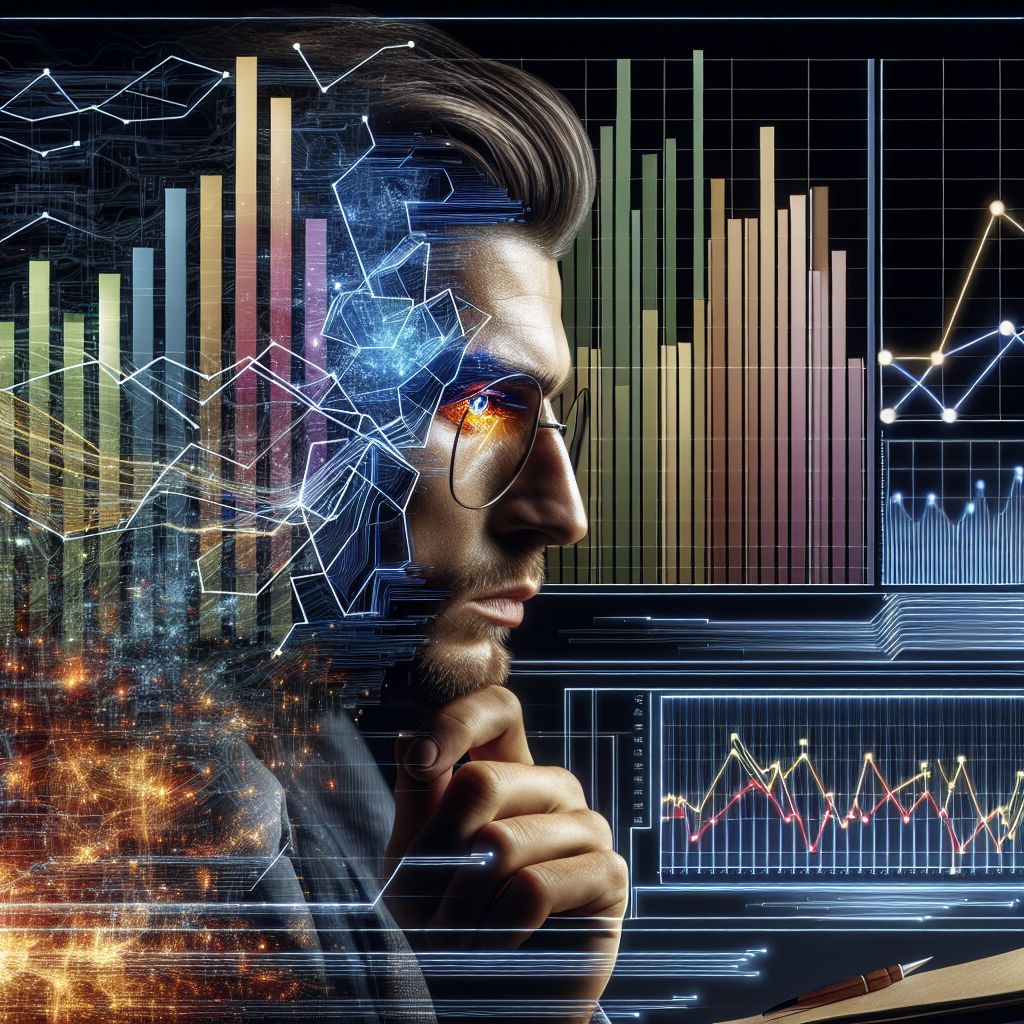
Why Use Python for Volume Profiles?
Python is a versatile programming language widely used in data analysis and quantitative finance. Its powerful libraries, such as Pandas and NumPy, make it ideal for financial data manipulation. Moreover, Python allows for easy automation and visualization, which are crucial for analyzing volume profiles.
Basic Python Setup
Before diving into the computation of volume profiles, ensure you have Python installed. Use Python.org to download the latest version.
pip install pandas numpy matplotlib
These libraries are essential for data manipulation and visualization.
Understanding Volume Profiles
Components of a Volume Profile
A volume profile consists of several components, each offering unique insights:
- Price Levels: Horizontal lines representing different prices.
- Volume Bars: Vertical bars indicating the volume traded at each price level.
- Point of Control (POC): The price level with the highest trading volume.
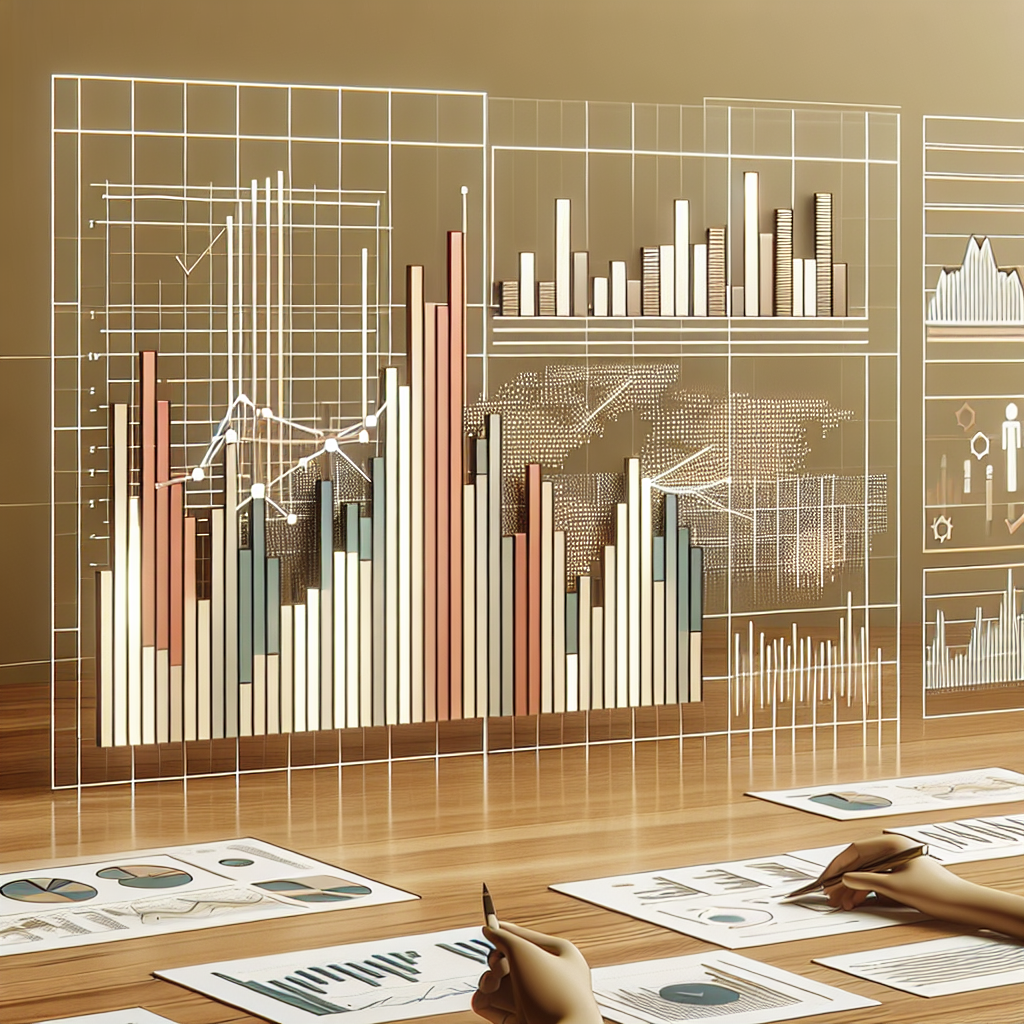
How Volume Profiles Aid in Trading
Volume profiles help traders identify crucial trading spots. For instance:
- Support and Resistance: Levels where the price tends to stay for extended periods.
- Market Trends: Volume peaks can indicate strong market sentiment.
Historical Context and Evolution
Volume profiles have evolved from traditional bar charts. Initially, traders used tick charts and candlesticks. The introduction of computerized trading made it easier to track and analyze large volumes of data, paving the way for more sophisticated tools like volume profiles.
Computing Volume Profiles in Python
Data Collection and Preparation
To compute volume profiles, you need historical price and volume data. You can use various sources like Yahoo Finance or Alpha Vantage. Below is an example of fetching data using Yahoo Finance:
import yfinance as yf
data = yf.download('AAPL', start='2022-01-01', end='2022-12-31')
prices = data['Close']
volume = data['Volume']
Volume Profile Calculation
The main objective is to aggregate the volume data at different price levels. You can achieve this using the Pandas library:
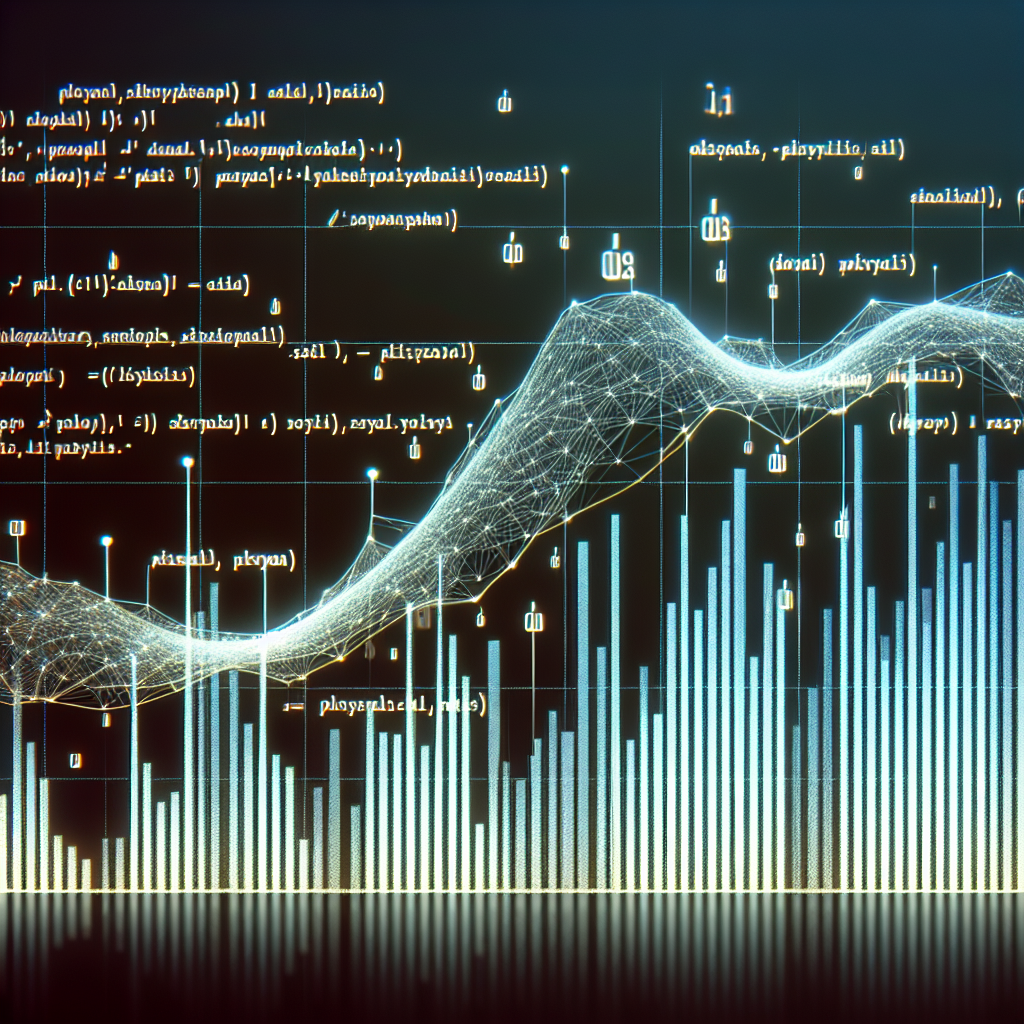
import pandas as pd
import numpy as np
def compute_volume_profile(prices, volume, bins=100):
hist, bin_edges = np.histogram(prices, bins=bins, weights=volume)
return hist, bin_edges
vol_profile, price_levels = compute_volume_profile(prices, volume)
Visualization of Volume Profiles
Visualization helps make sense of the computed data. Matplotlib is an excellent choice for this:
import matplotlib.pyplot as plt
plt.barh(price_levels[:-1], vol_profile, height=np.diff(price_levels), align='edge')
plt.xlabel('Volume')
plt.ylabel('Price Level')
plt.title('Volume Profile')
plt.show()
Practical Examples in Python
Example 1: Interactive Volume Profile with Bokeh
To make your volume profiles interactive, consider using Bokeh:
from bokeh.plotting import figure, show, output_file
from bokeh.io import output_notebook
output_notebook()
p = figure(y_range=(price_levels[0], price_levels[-1]), title="Interactive Volume Profile")
p.hbar(y=price_levels[:-1], right=vol_profile, left=0, height=np.diff(price_levels), color="navy")
show(p)
This provides an interactive visualization, enabling users to zoom and explore data dynamically.
Example 2: Volume Profile with Different Aggregation Levels
Sometimes, you might need a more granular view. Adjusting the bin size can help:
vol_profile_50, price_levels_50 = compute_volume_profile(prices, volume, bins=50)
plt.barh(price_levels_50[:-1], vol_profile_50, height=np.diff(price_levels_50), align='edge')
plt.xlabel('Volume')
plt.ylabel('Price Level')
plt.title('Volume Profile with 50 Bins')
plt.show()
Increasing or decreasing the number of bins provides a more or less detailed view, depending on the data granularity needed.
Advanced Techniques and Tips
Incorporating Moving Averages
Incorporating moving averages can provide additional context:
data['20_SMA'] = data['Close'].rolling(window=20).mean()
vol_profile_sma, sma_levels = compute_volume_profile(data['20_SMA'].dropna(), data['Volume'].iloc[19:])
plt.barh(sma_levels[:-1], vol_profile_sma, height=np.diff(sma_levels), align='edge')
plt.xlabel('Volume')
plt.ylabel('SMA Price Level')
plt.title('Volume Profile with 20-SMA')
plt.show()
Combining Volume Profiles with Other Indicators
Combining volume profiles with indicators like Relative Strength Index (RSI) or Bollinger Bands can yield powerful insights. For example:
import talib
data['RSI'] = talib.RSI(data['Close'], timeperiod=14)
high_rsi_zones = data[data['RSI'] > 70]['Close']
rsi_vol_profile, rsi_levels = compute_volume_profile(high_rsi_zones, data.loc[high_rsi_zones.index, 'Volume'])
plt.barh(rsi_levels[:-1], rsi_vol_profile, height=np.diff(rsi_levels), align='edge')
plt.xlabel('Volume')
plt.ylabel('High RSI Price Level')
plt.title('Volume Profile with High RSI Zones')
plt.show()
Real-World Application
Using volume profiles, traders can make more informed decisions. For example:
- Identifying Breakout Points: Spotting when the market is about to break through a resistance level.
- Volume Clusters: Recognizing areas of high trading activity to gauge market strength.
Conclusion
Recap of Key Points
- Volume profiles provide a detailed view of trading activity.
- Python's Pandas and Matplotlib libraries make it easy to compute and visualize volume profiles.
- Advanced techniques like incorporating moving averages or combining with other indicators enhance the analysis.
Practical Applications
Volume profiles are not just for academic purposes. Traders and analysts use them daily to make informed decisions about market behavior.
Final Thoughts
By mastering volume profiles in Python, you can significantly enhance your data analytical skills. The ability to visualize and interpret trading data opens up new avenues for research and trading strategies.
FAQ
What is a volume profile?
A volume profile is a chart that shows the trading activity (volume) at different price levels over a specified period.
Why use Python for volume profiles?
Python offers powerful libraries like Pandas and Matplotlib for data manipulation and visualization, making it ideal for financial analysis.
How do I get started with volume profiles in Python?
Start by installing the necessary libraries (Pandas
, NumPy
, Matplotlib
). Collect historical price and volume data, compute the volume profile, and visualize it.
Can I combine volume profiles with other indicators?
Yes, combining volume profiles with indicators like moving averages or RSI can provide deeper insights into market behavior.
Where can I find more resources on this topic?
Visit DataCamp Blog for tutorials and detailed articles on data analysis with Python.